I have heard about many interesting things about programming in Rust and I decided to use Rust for one of my applications. I enjoyed the programming experience in Rust and using the combination of Rust and Docker is quite interesting.
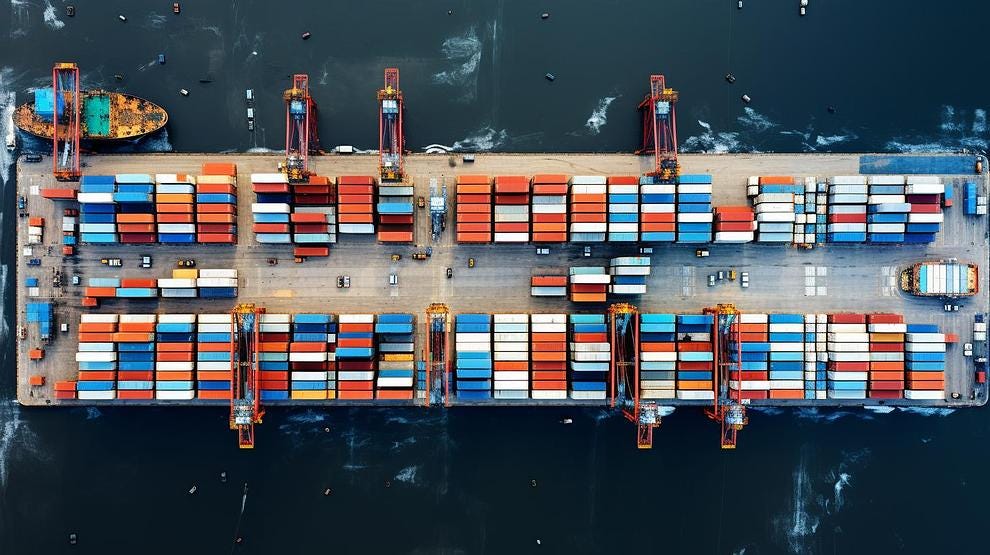
Rust is a system programming language that is popularly known for its memory safety, performance, and speed. Docker is a platform that enables developers to develop, deploy, and manage applications by using containerization. The combination of Docker and Rust creates an application that is both highly efficient and easily portable.
This article shows how to Dockerize your first application in Rust and deploy this application to AWS ECR. This is an assumption that you already have an understanding of AWS ECR and GitHub Actions.
Below is a step-by-step guide on how to Dockerize an application in Rust and deploy this application to AWS ECR.
Step 1: Setting Up Your Environment: Install Rust and Docker
Firstly, I ensure that both Docker and Rust are installed in your development environment. Run the following commands in your terminal to install Docker and Rust. The command installed rustup which helps to install the latest stable version of Rust.
# To install Rust
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
# To install Docker
# Follow this link https://docs.docker.com/engine/install/
Step 2: Setting Up the Project
Next, create a new Rust project using cargo Rust’s package manager and build system. Run the following command below. This will create a new directory rust_docker_guide
cargo new rust_docker_guide
cd rust_docker_guide
This will create a new directory named “rust_docker” with a basic Rust application. The main source file is located at src/main.rs. Open the file and you'll see the following code:
Step 3: Creating a Rust Application
Create the following code below in src/main.rs This is a simple Hello, world application in Rust Programming Language.
fn main() {
println!("Hello, world!");
}
Step 4: Creating the Dockerfile
To containerize the Rust Application, you need to create a Dockerfile . The Dockerfile contains instructions on how to build the Docker image of your application. Create a new file in the root directory of your project and name it Dockerfile , you can also use this command below to create the Dockerfile
touch Dockerfile
Open the Dockerfile and add the following content below:
# Use the official Rust image as the build environment
FROM rust:1.64 as builder
# Set the working directory
WORKDIR /usr/src/myapp
# Copy the Cargo.toml and Cargo.lock files
COPY Cargo.toml Cargo.lock ./
# Copy the source code
COPY src ./src
# Build the application in release mode
RUN cargo build --release
# Use a minimal base image to run the application
FROM debian:buster-slim
# Copy the build artifact from the builder stage
COPY --from=builder /usr/src/myapp/target/release/my_rust_app /usr/local/bin/my_rust_app
# Expose the application port
EXPOSE 8080
# Run the application
CMD ["my_rust_app"]
Step 5: Building and Running the Docker Image
With our Dockerfile ready, you can create the Docker Image for our Rust Application by executing the following command.
docker build -t rust_docker_guide:v0.0.1 .
This command will create a Docker image named rust_docker_guide
Step 6: Test the Dockerize Rust Application locally
This command will start the docker container and map port 8080 of the host machine to port 8080 of the container.
docker run -p 8080:8080 rust_docker_guide:v0.0.1
You can access the Rust App on your browser by running http://localhost:8080 and it will display Hello, World! confirming that the application is running successfully inside the Docker Container
Step 7: Authenticate with AWS
Before pushing your Docker image to Amazon ECR, you must authenticate with AWS services using either IAM roles or AWS access keys and secret keys.
Step 8: Create AWS ECR Repository
You will need to create a AWS ECR Repository. Use the command below to create the repository. Replace <your_account_id>.dkr.ecr.us-east-1.amazonaws.com with your newly created Amazon ECR URI
aws ecr create-repository --repository-name rust_docker_guide
aws ecr get-login-password --region us-east-1 | docker login --username AWS --password-stdin <your_account_id>.dkr.ecr.us-east-1.amazonaws.com
Step 9: Push Docker Image to Amazon ECR
The next step is to push the docker image you built locally to AWS ECR. See the command below on how to achieve this. Replace <your_account_id> with your AWS Account
docker tag rust_docker_guide:v0.0.1 <your_account_id>.dkr.ecr.us-east-1.amazonaws.com/rust_docker_guide:v0.0.1
docker push <your_account_id>.dkr.ecr.us-east-1.amazonaws.com/rust_docker_guide:v0.0.1
Conclusion
I hope you find this process of dockerizing Rust Application useful and interesting. Check out the complete code on GitHub